Sealed Classes in Java
Unlocking the Power of Java 17’s Sealed Classes

Introduction:
Java, one of the most powerful programming languages, has consistently evolved to meet the needs of modern developers. With each new version, it introduces new features that improve efficiency, readability, and security. One such feature is the Sealed Classes introduced in Java 17.
What are Sealed Classes?
In simpler terms, sealed classes give you more control over inheritance. By using sealed classes, you can define a class or interface that restricts which other classes or interfaces can extend or implement it. This feature provides a way to maintain a closed set of subtypes, thus ensuring that only specific, known types can extend or implement the class.
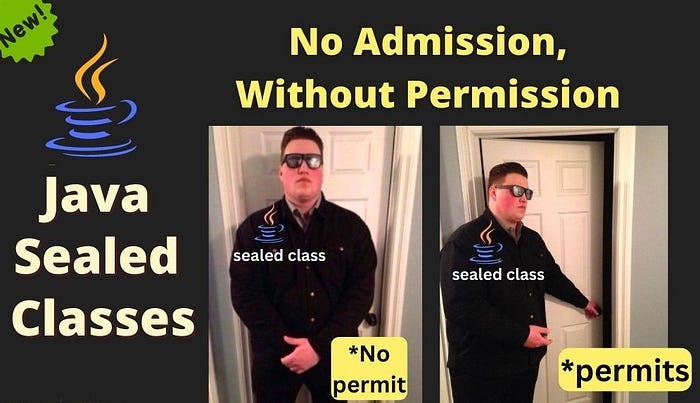
Why Sealed Classes Matter:
Before Java 17, developers could use regular inheritance to create subclasses from any class. While this approach offered flexibility, it also introduced potential risks. For example, you could unintentionally create subclasses in other packages or third-party libraries that may break the functionality or violate business logic.
With sealed classes, Java now lets you explicitly define the allowed subclasses. This can help in:
- Ensuring a closed set of subclasses: Only the defined classes or interfaces are allowed to extend or implement the sealed class.
- Improving maintainability: You can easily manage which classes are part of a particular hierarchy, making your codebase easier to maintain.
- Better security: By limiting the inheritance of your classes, you reduce the chances of unintended subclassing, which can introduce bugs and vulnerabilities.
Syntax of Sealed Classes:
Here’s how you can define a sealed class:
public sealed class Animal
permits Dog, Cat { // Only Dog and Cat can extend Animal
}
In this example, the Animal
class is sealed, and only the classes Dog
and Cat
are allowed to extend it. Any attempt to extend Animal
with other classes outside of the permits
list will result in a compilation error.
Sealed Interfaces:
Sealed classes can also be used in interfaces, where you can control which classes or interfaces can implement your interface.
Here’s an example:
public sealed interface Shape
permits Circle, Rectangle { // Only Circle and Rectangle can implement Shape
}
public final class Circle implements Shape {}
public final class Rectangle implements Shape {}
Benefits of Sealed Classes:
- Better Design and Encapsulation: Sealed classes allow developers to better define the scope of their APIs, improving code encapsulation. By using the
permits
clause, you can define which classes are allowed to extend your class or interface, and prevent external modifications. - Performance Optimization: Since the Java compiler knows all the subclasses upfront, it can perform certain optimizations in methods like
switch
statements. This reduces runtime overhead and improves performance. - Clearer Intent: When you mark a class or interface as sealed, you are making a statement about the design of your system. This helps other developers understand that this is a carefully structured class hierarchy that’s not meant to be extended beyond the predefined types.
Sealed Class Restrictions:
While the feature offers several advantages, it also comes with some restrictions:
- A sealed class cannot be extended or implemented by other classes unless explicitly stated in the
permits
clause. - You cannot mark a class as both sealed and
abstract
. Sealed classes can either be fully implemented or have specific subclasses, but they cannot be used as templates for multiple subclasses.
Real-World Use Cases:
- Modeling Restricted Domain Models: When you have a set of known, limited types, such as a set of payment methods (
CreditCard
,DebitCard
,PayPal
), sealed classes can ensure that no other types can accidentally extend them. - State Machines: For state transitions, sealed classes can help by ensuring that only predefined states are possible, enhancing code clarity and reducing errors.
Conclusion:
Sealed classes are a powerful addition to Java, providing a way to limit inheritance and improve code safety, maintainability, and performance. Whether you’re working on complex systems with strict type hierarchies or trying to ensure security and clarity in your domain models, sealed classes give you the control and structure that traditional inheritance could never achieve.
If you’re using Java 17 or later, it’s worth exploring sealed classes to better design your applications and take advantage of this modern Java feature.
🌟 Encouragement & Interaction 🌟
If you found this article informative and helpful, please consider expressing your appreciation by giving it a clap 👏. Don’t hesitate to share this article with your colleagues. Your support and sharing of knowledge within the developer community are greatly appreciated.
👉 Please share on social media
👉 Follow me on : Medium || LinkedIn